Usage#
Installation#
Install from PyPI:
$ pip install django-relatives
Linking to foreign keys in change lists#
The easiest way to automatically to turn foreign key columns into hyperlinks in a Django admin change list is to inherit from RelativesAdmin
in your model admin.
Example#
from django.contrib import admin
from relatives import RelativesAdmin
from .models import Company, Employee
@admin.register(Company)
class CompanyAdmin(admin.ModelAdmin):
list_display = ["name"]
@admin.register(Employee)
class EmployeeAdmin(RelativesAdmin):
list_display = ["first_name", "last_name", "company"]
Screenshot#
When viewing the change list for the employee model, you’ll now see that the company column contents are all linked to the appropriate change form page for each company.
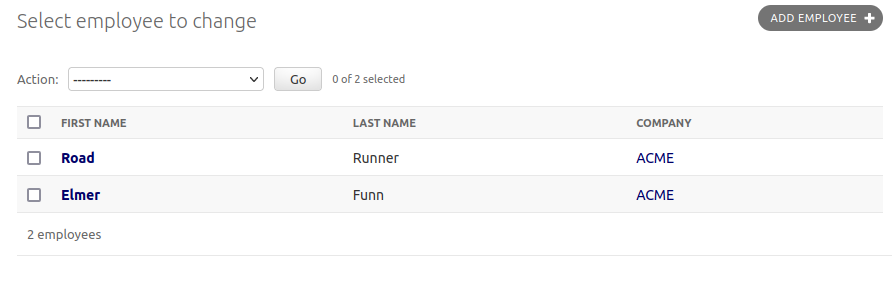
Linking to foreign keys in change forms#
The contents_or_fk_link
template filter can be used to link to foreign keys for readonly admin form fields.
Django Relatives also provides a replacement for the admin/includes/fieldset.html
template which can be used to automatically link to all readonly foreign key fields in change forms.
To use the custom fieldset template you must add relatives
to
INSTALLED_APPS
in your settings file:
INSTALLED_APPS = (
...
'relatives',
)
Next create a admin/includes/fieldset.html
template file:
{% extends "relatives/includes/fieldset.html" %}
Also make sure this template file is in a custom template directory or an app
listed before your admin app in INSTALLED_APPS
.
Example Screenshot#
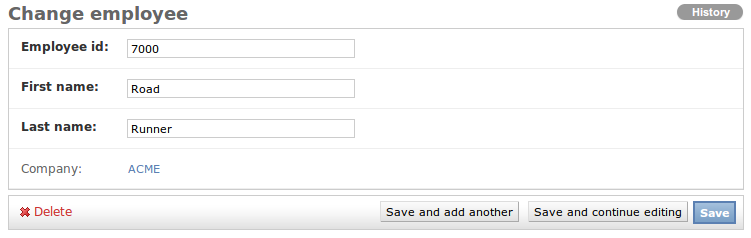
Linking to reverse relations on change forms#
The related_objects
template tag makes it easy to link to change lists
filtered for reverse relations (objects that have a foreign key to a given
object).
Django Relatives also provides a custom change_form.html
template that may
be used to add a “Relations” sidebar to change forms. This sidebar provides
links to change list queries for all objects that contain a foreign key to the
current object.
To use the custom fieldset template you must add relatives
to
INSTALLED_APPS
in your settings file:
INSTALLED_APPS = (
...
'relatives',
)
Now you can customize the change form template for your desired models/apps.
The easiest way to link to reverse relations is to override the
change_form_template
in your ModelAdmin
subclass.
Example#
Code#
from django.contrib import admin
from .models import Company, Employee
admin.site.register(Employee)
@admin.register(Company, CompanyAdmin)
class CompanyAdmin(admin.ModelAdmin):
change_form_template = "relatives/change_form.html"
Screenshot#

Linking to reverse relations with custom template#
If you don’t have access to change the ModelAdmin
for your model or you are
already customizing your model’s admin change form, you will need to use a
custom admin template instead.
Create a admin/YOURAPP/YOURMODEL/change_form.html
template file that
extends from relatives/change_form.html
:
{% extends "relatives/change_form.html" %}
Also make sure this template file is in a custom template directory or an app
listed before your admin app in INSTALLED_APPS
.
Edit links in inlines#
To link to an inline object, include the object_link
utility function in your admin inline’s fields
list and readonly_fields
list.
Example#
Code#
from django.contrib import admin
from relatives.utils import object_link
from .models import Company, Employee
admin.site.register(Employee)
class EmployeeInline(admin.TabularInline):
model = Employee
fields = [object_link, "first_name", "last_name"]
readonly_fields = fields
extra = 0
max_num = 0
can_delete = False
@admin.register(Company)
class CompanyAdmin(admin.ModelAdmin):
inlines = [EmployeeInline]
Screenshot#
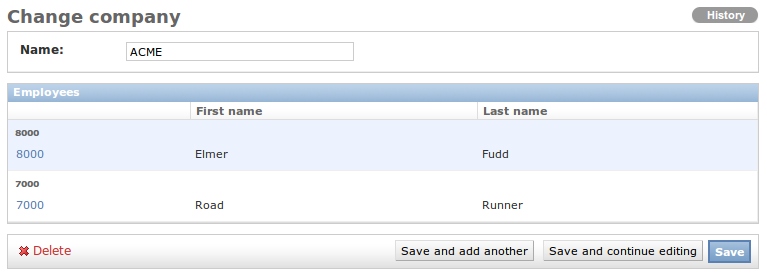
Customizing inline edit links#
To customize the link text for your inline links, use the object_edit_link
utility function instead, specifying the edit text and blank text (both are optional).
Example#
Code#
from django.contrib import admin
from relatives.utils import object_edit_link
from .models import Company, Employee
admin.site.register(Employee)
class EmployeeInline(admin.TabularInline):
model = Employee
edit_link = object_edit_link("Edit")
fields = [edit_link, "employee_id", "first_name", "last_name"]
readonly_fields = [edit_link]
@admin.register(Company)
class CompanyAdmin(admin.ModelAdmin):
inlines = [EmployeeInline]
Screenshot#
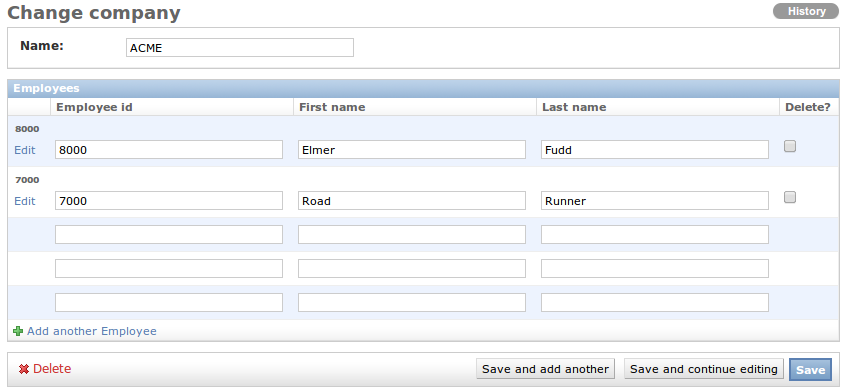
Customizing settings#
Since relatives uses cache, you can update the settings module to change the defaults if you’d like:
RELATIVES_CACHE_KEY = 'relatives_cache'
RELATIVES_CACHE_TIME = int(60*60*24)